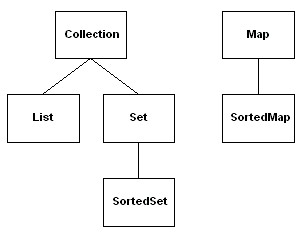
Collection is a major part of the Java programming language. It is difficult to learn, but it pays once you are familiar with them. The following short articles use simple examples to explain the key ideas.
Java Collections
- Collections Hierarchy Diagram
- Collection classes summary table
- Set: HashSet vs. TreeSet vs. LinkedHashSet
- List: ArrayList vs. LinkedList vs. Vector
- Map: HashMap vs. TreeMap vs. Hashtable vs. LinkedHashMap
- Top 10 questions about Java Collections
- Top 9 questions about Java Maps
- Make an efficient counter using HashMap
- Set vs. Set<?>
- java.util.ConcurrentModificationException
More Code Examples
- 3 frequently used methods for HashMap
- Set for a Collection of Unique Data
- TreeSet – Simple Example
- TreeSet – Implement Comparable
- PriorityQueue
- Sorting Arrays
- Sort a linked list of self-defined objects
- Convert Hashtable to TreeMap
Generics
- Why do we need generic types in Java?
- Why do we need generic methods?
- Generic Types vs. Generic Methods
- Java Type Erasure Mechanism
- 3 examples to explain common mistakes when using Java Generics
There are more Java API Examples from programcreek.