Java Code Examples for io.noties.markwon.Markwon#setMarkdown()
The following examples show how to use
io.noties.markwon.Markwon#setMarkdown() .
You can vote up the ones you like or vote down the ones you don't like,
and go to the original project or source file by following the links above each example. You may check out the related API usage on the sidebar.
Example 1
Source File: CustomExtensionActivity.java From Markwon with Apache License 2.0 | 6 votes |
@Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_text_view); final TextView textView = findViewById(R.id.text_view); // note that we haven't registered CorePlugin, as it's the only one that can be // implicitly deducted and added automatically. All other plugins require explicit // `usePlugin` call final Markwon markwon = Markwon.builder(this) // try commenting out this line to see runtime dependency resolution // .usePlugin(ImagesPlugin.create(this)) .usePlugin(IconPlugin.create(IconSpanProvider.create(this, 0))) .build(); markwon.setMarkdown(textView, getString(R.string.input)); }
Example 2
Source File: TableActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void withLatex() { String latex = "\\begin{array}{cc}"; latex += "\\fbox{\\text{A framed box with \\textdbend}}&\\shadowbox{\\text{A shadowed box}}\\cr"; latex += "\\doublebox{\\text{A double framed box}}&\\ovalbox{\\text{An oval framed box}}\\cr"; latex += "\\end{array}"; final String md = "" + "| HEADER | HEADER |\n" + "|:----:|:----:|\n" + "|  | Build |\n" + "| Stable |  |\n" + "| BIG | $$" + latex + "$$ |\n" + "\n"; final Markwon markwon = Markwon.builder(this) .usePlugin(MarkwonInlineParserPlugin.create()) .usePlugin(ImagesPlugin.create()) .usePlugin(JLatexMathPlugin.create(textView.getTextSize(), builder -> builder.inlinesEnabled(true))) .usePlugin(TablePlugin.create(this)) .build(); markwon.setMarkdown(textView, md); }
Example 3
Source File: HtmlActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void randomCharSize() { final String md = "" + "<random-char-size>\n" + "This message should have a jumpy feeling because of different sizes of characters\n" + "</random-char-size>\n\n"; final Markwon markwon = Markwon.builder(this) .usePlugin(HtmlPlugin.create()) .usePlugin(new AbstractMarkwonPlugin() { @Override public void configure(@NonNull Registry registry) { registry.require(HtmlPlugin.class, htmlPlugin -> htmlPlugin .addHandler(new RandomCharSize(new Random(42L), textView.getTextSize()))); } }) .build(); markwon.setMarkdown(textView, md); }
Example 4
Source File: TableActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void tableAndLinkify() { final String md = "" + "| HEADER | HEADER | HEADER |\n" + "|:----:|:----:|:----:|\n" + "| 测试 | 测试 | 测试 |\n" + "| 测试 | 测试 | 测测测12345试测试测试 |\n" + "| 测试 | 测试 | 123445 |\n" + "| 测试 | 测试 | (650) 555-1212 |\n" + "| 测试 | 测试 | [link](#) |\n" + "\n" + "测试\n" + "\n" + "[link link](https://link.link)"; final Markwon markwon = Markwon.builder(this) .usePlugin(LinkifyPlugin.create()) .usePlugin(TablePlugin.create(this)) .build(); markwon.setMarkdown(textView, md); }
Example 5
Source File: TableActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void customize() { final String md = "" + "| HEADER | HEADER | HEADER |\n" + "|:----:|:----:|:----:|\n" + "| 测试 | 测试 | 测试 |\n" + "| 测试 | 测试 | 测测测12345试测试测试 |\n" + "| 测试 | 测试 | 123445 |\n" + "| 测试 | 测试 | (650) 555-1212 |\n" + "| 测试 | 测试 | [link](#) |\n"; final Markwon markwon = Markwon.builder(this) .usePlugin(TablePlugin.create(builder -> { final Dip dip = Dip.create(this); builder .tableBorderWidth(dip.toPx(2)) .tableBorderColor(Color.YELLOW) .tableCellPadding(dip.toPx(4)) .tableHeaderRowBackgroundColor(ColorUtils.applyAlpha(Color.RED, 80)) .tableEvenRowBackgroundColor(ColorUtils.applyAlpha(Color.GREEN, 80)) .tableOddRowBackgroundColor(ColorUtils.applyAlpha(Color.BLUE, 80)); })) .build(); markwon.setMarkdown(textView, md); }
Example 6
Source File: HtmlActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void align() { final String md = "" + "<align center>We are centered</align>\n" + "\n" + "<align end>We are at the end</align>\n" + "\n" + "<align>We should be at the start</align>\n" + "\n"; final Markwon markwon = Markwon.builder(this) .usePlugin(HtmlPlugin.create()) .usePlugin(new AbstractMarkwonPlugin() { @Override public void configure(@NonNull Registry registry) { registry.require(HtmlPlugin.class, htmlPlugin -> htmlPlugin .addHandler(new AlignTagHandler())); } }) .build(); markwon.setMarkdown(textView, md); }
Example 7
Source File: BasicPluginsActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void tableOfContents() { final String lorem = getString(R.string.lorem); final String md = "" + "# First\n" + "" + lorem + "\n\n" + "# Second\n" + "" + lorem + "\n\n" + "## Second level\n\n" + "" + lorem + "\n\n" + "### Level 3\n\n" + "" + lorem + "\n\n" + "# First again\n" + "" + lorem + "\n\n"; final Markwon markwon = Markwon.builder(this) .usePlugin(new TableOfContentsPlugin()) .usePlugin(new AnchorHeadingPlugin((view, top) -> scrollView.smoothScrollTo(0, top))) .build(); markwon.setMarkdown(textView, md); }
Example 8
Source File: BasicPluginsActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void letterOrderedList() { // bullet list nested in ordered list renders letters instead of bullets final String md = "" + "1. Hello there!\n" + "1. And here is how:\n" + " - First\n" + " - Second\n" + " - Third\n" + " 1. And first here\n\n"; final Markwon markwon = Markwon.builder(this) .usePlugin(new BulletListIsOrderedWithLettersWhenNestedPlugin()) .build(); markwon.setMarkdown(textView, md); }
Example 9
Source File: InlineParserActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void pluginNoDefaults() { // a plugin with NO defaults registered final String md = "no [links](#) for **you** `code`!"; final Markwon markwon = Markwon.builder(this) // pass `MarkwonInlineParser.factoryBuilderNoDefaults()` no disable all .usePlugin(MarkwonInlineParserPlugin.create(MarkwonInlineParser.factoryBuilderNoDefaults())) .usePlugin(new AbstractMarkwonPlugin() { @Override public void configure(@NonNull Registry registry) { registry.require(MarkwonInlineParserPlugin.class, plugin -> { plugin.factoryBuilder() .addInlineProcessor(new BackticksInlineProcessor()); }); } }) .build(); markwon.setMarkdown(textView, md); }
Example 10
Source File: InlineParserActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void pluginWithDefaults() { // a plugin with defaults registered final String md = "no [links](#) for **you** `code`!"; final Markwon markwon = Markwon.builder(this) .usePlugin(MarkwonInlineParserPlugin.create()) // the same as: // .usePlugin(MarkwonInlineParserPlugin.create(MarkwonInlineParser.factoryBuilder())) .usePlugin(new AbstractMarkwonPlugin() { @Override public void configure(@NonNull Registry registry) { registry.require(MarkwonInlineParserPlugin.class, plugin -> { plugin.factoryBuilder() .excludeInlineProcessor(OpenBracketInlineProcessor.class); }); } }) .build(); markwon.setMarkdown(textView, md); }
Example 11
Source File: HtmlActivity.java From Markwon with Apache License 2.0 | 6 votes |
private void enhance() { final String md = "" + "<enhance start=\"5\" end=\"12\">This is text that must be enhanced, at least a part of it</enhance>"; final Markwon markwon = Markwon.builder(this) .usePlugin(HtmlPlugin.create()) .usePlugin(new AbstractMarkwonPlugin() { @Override public void configure(@NonNull Registry registry) { registry.require(HtmlPlugin.class, htmlPlugin -> htmlPlugin .addHandler(new EnhanceTagHandler((int) (textView.getTextSize() * 2 + .05F)))); } }) .build(); markwon.setMarkdown(textView, md); }
Example 12
Source File: BasicPluginsActivity.java From Markwon with Apache License 2.0 | 5 votes |
private void softBreakAddsNewLine() { // insert a new line when markdown has a soft break final Markwon markwon = Markwon.builder(this) .usePlugin(SoftBreakAddsNewLinePlugin.create()) .build(); final String md = "" + "Hello there ->(line)\n(break)<- going on and on"; markwon.setMarkdown(textView, md); }
Example 13
Source File: BasicPluginsActivity.java From Markwon with Apache License 2.0 | 5 votes |
private void anchor() { final String lorem = getString(R.string.lorem); final String md = "" + "Hello [there](#there)!\n\n\n" + lorem + "\n\n" + "# There!\n\n" + lorem; final Markwon markwon = Markwon.builder(this) .usePlugin(new AnchorHeadingPlugin((view, top) -> scrollView.smoothScrollTo(0, top))) .build(); markwon.setMarkdown(textView, md); }
Example 14
Source File: TaskListActivity.java From Markwon with Apache License 2.0 | 5 votes |
private void customDrawableResources() { // drawable **must** be stateful final Drawable drawable = Objects.requireNonNull( ContextCompat.getDrawable(this, R.drawable.custom_task_list)); final Markwon markwon = Markwon.builder(this) .usePlugin(TaskListPlugin.create(drawable)) .build(); markwon.setMarkdown(textView, MD); }
Example 15
Source File: LatexActivity.java From Markwon with Apache License 2.0 | 5 votes |
private void legacy() { final String md = wrapLatexInSampleMarkdown(LATEX_BANGLE); final Markwon markwon = Markwon.builder(this) // LEGACY does not require inline parser .usePlugin(JLatexMathPlugin.create(textView.getTextSize(), builder -> { builder.blocksLegacy(true); builder.theme() .backgroundProvider(() -> new ColorDrawable(0x100000ff)) .padding(JLatexMathTheme.Padding.all(48)); })) .build(); markwon.setMarkdown(textView, md); }
Example 16
Source File: HtmlActivity.java From Markwon with Apache License 2.0 | 5 votes |
private void iframe() { final String md = "" + "# Hello iframe\n\n" + "<p class=\"p1\"><img title=\"JUMP FORCE\" src=\"https://img1.ak.crunchyroll.com/i/spire1/f0c009039dd9f8dff5907fff148adfca1587067000_full.jpg\" alt=\"JUMP FORCE\" width=\"640\" height=\"362\" /></p>\n" + "<p class=\"p2\"> </p>\n" + "<p class=\"p1\">Switch owners will soon get to take part in the ultimate <em>Shonen Jump </em>rumble. Bandai Namco announced plans to bring <strong><em>Jump Force </em></strong>to <strong>Switch</strong> as <strong><em>Jump Force Deluxe Edition</em></strong>, with a release set for sometime this year. This version will include all of the original playable characters and those from Character Pass 1, and <strong>Character Pass 2 is also in the works </strong>for all versions, starting with <strong>Shoto Todoroki from </strong><span style=\"color: #ff9900;\"><a href=\"/my-hero-academia?utm_source=editorial_cr&utm_medium=news&utm_campaign=article_driven&referrer=editorial_cr_news_article_driven\"><span style=\"color: #ff9900;\"><strong><em>My Hero Academia</em></strong></span></a></span>.</p>\n" + "<p class=\"p2\"> </p>\n" + "<p class=\"p1\">Other than Todoroki, Bandai Namco hinted that the four other Character Pass 2 characters will hail from <span style=\"color: #ff9900;\"><a href=\"/hunter-x-hunter?utm_source=editorial_cr&utm_medium=news&utm_campaign=article_driven&referrer=editorial_cr_news_article_driven\"><span style=\"color: #ff9900;\"><em>Hunter x Hunter</em></span></a></span>, <em>Yu Yu Hakusho</em>, <span style=\"color: #ff9900;\"><a href=\"/bleach?utm_source=editorial_cr&utm_medium=news&utm_campaign=article_driven&referrer=editorial_cr_news_article_driven\"><span style=\"color: #ff9900;\"><em>Bleach</em></span></a></span>, and <span style=\"color: #ff9900;\"><a href=\"/jojos-bizarre-adventure?utm_source=editorial_cr&utm_medium=news&utm_campaign=article_driven&referrer=editorial_cr_news_article_driven\"><span style=\"color: #ff9900;\"><em>JoJo's Bizarre Adventure</em></span></a></span>. Character Pass 2 will be priced at $17.99, and Todoroki launches this spring.<span class=\"Apple-converted-space\"> </span></p>\n" + "<p class=\"p2\"> </p>\n" + "<p class=\"p1\"><iframe style=\"display: block; margin-left: auto; margin-right: auto;\" src=\"https://www.youtube.com/embed/At1qTj-LWCc\" frameborder=\"0\" width=\"640\" height=\"360\"></iframe></p>\n" + "<p class=\"p2\"> </p>\n" + "<p class=\"p1\">Character Pass 2 promo:</p>\n" + "<p class=\"p2\"> </p>\n" + "<p class=\"p1\"><iframe style=\"display: block; margin-left: auto; margin-right: auto;\" src=\"https://www.youtube.com/embed/CukwN6kV4R4\" frameborder=\"0\" width=\"640\" height=\"360\"></iframe></p>\n" + "<p class=\"p2\"> </p>\n" + "<p class=\"p1\"><a href=\"https://got.cr/PremiumTrial-NewsBanner4\"><img style=\"display: block; margin-left: auto; margin-right: auto;\" src=\"https://img1.ak.crunchyroll.com/i/spire4/78f5441d927cf160a93e037b567c2b1f1587067041_full.png\" alt=\"\" width=\"640\" height=\"43\" /></a></p>\n" + "<p class=\"p2\"> </p>\n" + "<p class=\"p1\">-------</p>\n" + "<p class=\"p1\"><em>Joseph Luster is the Games and Web editor at </em><a href=\"http://www.otakuusamagazine.com/ME2/Default.asp\"><em>Otaku USA Magazine</em></a><em>. You can read his webcomic, </em><a href=\"http://subhumanzoids.com/comics/big-dumb-fighting-idiots/\">BIG DUMB FIGHTING IDIOTS</a><em> at </em><a href=\"http://subhumanzoids.com/\"><em>subhumanzoids</em></a><em>. Follow him on Twitter </em><a href=\"https://twitter.com/Moldilox\"><em>@Moldilox</em></a><em>.</em><span class=\"Apple-converted-space\"> </span></p>"; final Markwon markwon = Markwon.builder(this) .usePlugin(ImagesPlugin.create()) .usePlugin(HtmlPlugin.create()) .usePlugin(new IFrameHtmlPlugin()) .build(); markwon.setMarkdown(textView, md); }
Example 17
Source File: ImagesActivity.java From Markwon with Apache License 2.0 | 5 votes |
private void glideSingleImageWithPlaceholder() { final String md = "[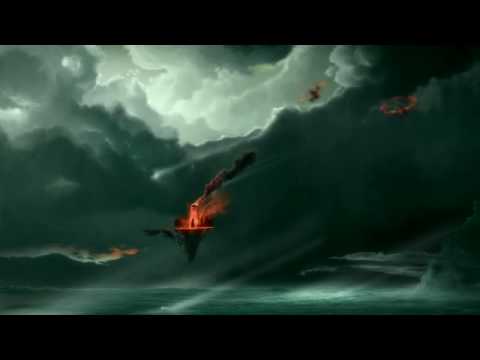](https://www.youtube.com/watch?v=gs1I8_m4AOM)"; final Context context = this; final Markwon markwon = Markwon.builder(context) .usePlugin(GlideImagesPlugin.create(new GlideImagesPlugin.GlideStore() { @NonNull @Override public RequestBuilder<Drawable> load(@NonNull AsyncDrawable drawable) { // final Drawable placeholder = ContextCompat.getDrawable(context, R.drawable.ic_home_black_36dp); // placeholder.setBounds(0, 0, 100, 100); return Glide.with(context) .load(drawable.getDestination()) // .placeholder(ContextCompat.getDrawable(context, R.drawable.ic_home_black_36dp)); // .placeholder(placeholder); .placeholder(R.drawable.ic_home_black_36dp); } @Override public void cancel(@NonNull Target<?> target) { Glide.with(context) .clear(target); } })) .build(); markwon.setMarkdown(textView, md); }
Example 18
Source File: CustomExtensionActivity2.java From Markwon with Apache License 2.0 | 5 votes |
private void text_added() { final Markwon markwon = Markwon.builder(this) .usePlugin(new AbstractMarkwonPlugin() { @Override public void configure(@NonNull Registry registry) { registry.require(CorePlugin.class, corePlugin -> corePlugin.addOnTextAddedListener(new GithubLinkifyRegexTextAddedListener())); } }) .build(); markwon.setMarkdown(textView, MD); }
Example 19
Source File: BasicPluginsActivity.java From Markwon with Apache License 2.0 | 4 votes |
/** * Images configuration. Can be used with (or without) ImagesPlugin, which does some basic * images handling (parsing markdown containing images, obtain an image from network * file system or assets). Please note that */ private void imagesPlugin() { final String markdown = ""; final Markwon markwon = Markwon.builder(this) .usePlugin(ImagesPlugin.create()) .usePlugin(new AbstractMarkwonPlugin() { @Override public void configure(@NonNull Registry registry) { // use registry.require to obtain a plugin, does also // a runtime validation if this plugin is registered registry.require(ImagesPlugin.class, plugin -> plugin.addSchemeHandler(new SchemeHandler() { // it's a sample only, most likely you won't need to // use existing scheme-handler, this for demonstration purposes only final NetworkSchemeHandler handler = NetworkSchemeHandler.create(); @NonNull @Override public ImageItem handle(@NonNull String raw, @NonNull Uri uri) { final String url = raw.replace("myownscheme", "https"); return handler.handle(url, Uri.parse(url)); } @NonNull @Override public Collection<String> supportedSchemes() { return Collections.singleton("myownscheme"); } })); } }) // or we can init plugin with this factory method // .usePlugin(ImagesPlugin.create(plugin -> { // plugin.addSchemeHandler(/**/) // })) .build(); markwon.setMarkdown(textView, markdown); }